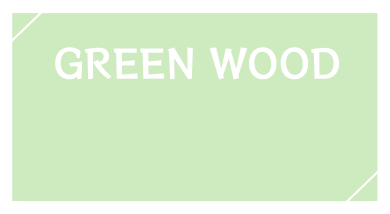
タイトル:芸術の秋 (11月)
使用言語:p5.js(Javascriptフレームワーク)
プログラミングコード
/**************
* カラータイル(container1)
**************/
let sketch1 = function(p1) {
//変数設定
let x,y;
let w,h;
let direction=0;
p1.setup = function() {
if(p1.windowWidth>=820){
p1.createCanvas(485,400);
}else if(p1.windowWidth < 820 && p1.windowWidth>=450){
p1.createCanvas(485,400);
}else if(p1.windowWidth < 450){
p1.createCanvas(200,200);
}
//p1.background(255);
x=0;
y=0;
w=p1.width;
h=p1.height;
p1.frameRate(1);
};
p1.draw = function() {
p1.clear ();
p1.fill(255);
divide(x, y, w, h, 0, direction);
//p1.noLoop();
};
//タイルの分割関数
function divide(x,y,w,h,count,direction){
let tiles=[];
let split =p1.random(0.2,0.8);
if(direction==0){
tiles.push(new Tile(x,y,w*split,h));
tiles.push(new Tile(x+w*split,y,w-w*split,h));
direction=1;
}else{
tiles.push(new Tile(x,y,w,h*split));
tiles.push(new Tile(x,y+h*split,w,h-h*split));
direction=0;
}
for (let i=0; i < tiles.length; i++) {
if (count < 5) {
divide(tiles[i].x, tiles[i].y, tiles[i].w, tiles[i].h, count + 1, direction);
} else {
tiles[i].show();
}
}
}
//タイルクラス(1枚のタイル)
class Tile {
constructor(x, y, w, h) {
this.x = x; //位置
this.y = y; //y位置
this.w = w; //幅
this.h = h; //高さ
this.a=50 //透明度
//タイルの色
this.c= [
[255,0,0,this.a],[255,255,0,this.a],[255,0,255,this.a],
[0,255,0,this.a],[0,255,255,this.a],[0,0,255,this.a]
];
}
//タイルの描画
show() {
p1.fill(p1.random(this.c));
p1.strokeWeight(3);
p1.stroke(0);
p1.rect(this.x, this.y, this.w, this.h);
}
}
};
new p5(sketch1, "container1");
/**************
* container2(フローフィールド)
**************/
var sketch2 = function(p) {
//変数設定
let cols,rows;
let size=50;
let arrows=[];
let r=size/2;
let xoff=0,yoff=0,zoff=0;
let increment=0.1;
let particles=[];
let num=100;
//ラインの色
let col=[
[255,0,0,100],[0,255,0,100],[0,0,255,100],
[255,0,255,100],[255,255,0,100],[0,255,255,100],
];
//画像変数
let img;
//絵描きの画像読み込み
p.preload=function(){
img=p.loadImage('ekaki.png');
}
p.setup = function() {
//キャンバス設定
if(p.windowWidth>=820){
p.createCanvas(400,400);
}else if(p.windowWidth < 820 && p.windowWidth>=450){
p.createCanvas(400,400);
}else if(p.windowWidth < 450){
p.createCanvas(200,200);
}
p.angleMode(p.DEGREES);
//升目
cols=p.width/size;
rows=p.height/size;
//インスタンス
for(let i=0;i < num;i++){
particles[i]=new Particle(p.random(0,p.width),p.random(0,p.height),col[i%col.length]);
}
p.background(50,50,50,100);
};
p.draw = function() {
p.fill(255);
xoff=0;
for (let i=0; i < cols; i++) {
arrows[i] = [];
yoff = 0;
for (let j=0; j < rows; j++) {
let angle = p.map(p.noise(xoff, yoff, zoff), 0, 1, 0, 360);
// p.rect(i*size,j*size,size,size);
//p.text(p.round(angle,2),size/2+i*size,size/2+j*size);
arrows[i][j]=p.createVector(p.cos(angle),p.sin (angle));
// let pt0 = p.createVector(size/2+ i*size, size/2+j*size);
// let pt1 = p.createVector(r*arrows[i][j].x, r*arrows[i][j].y);
// p.line(pt0.x, pt0.y, pt0.x + pt1.x, pt0.y + pt1.y);
// p.ellipse(pt0.x + pt1.x, pt0.y + pt1.y, 5, 5);
yoff+=increment;
}
xoff+=increment;
zoff+=0.001;
}
//フロー描画
for(let i=0;i < num;i++){
particles[i].display();
particles[i].update();
particles[i].direction(arrows);
particles[i].checkEdges();
}
//絵描きの配置
if(p.windowWidth>=820){
p.image(img,0,100);
}else if(p.windowWidth < 820 && p.windowWidth>=450){
p.image(img,0,100);
}else if(p.windowWidth < 450){
img.resize(100,100)
p.image(img,50,100);
}
}
//パーティクルクラス
class Particle{
constructor(x,y,col){
this.position=p.createVector(x,y);
this.velocity=p.createVector(0,0);
this.acceleration=p.createVector(0,0);
this.col=col;
}
update(){
this.velocity.add(this.acceleration);
this.velocity.limit(2); //ベクトルの大きさを最大値に制限
this.position.add(this.velocity);
this.acceleration.mult(0);
}
//作用する方向ベクトル
direction(flowfield){
let i=p.floor(this.position.x/size); //整数に
let j=p.floor(this.position.y/size); //整数に
i=p.constrain(i,0,cols-1); //iを0~cols-1の範囲に制限
j=p.constrain(j,0,rows-1);
let force=p.createVector(flowfield[i][j].x,flowfield[i][j].y); //方向ベクトル
this.applyForce(force)
}
applyForce(force){
this.acceleration.add(force); //加速の方向
}
//パーティクル描画
display(){
p.noStroke();
p.fill(this.col);
p.ellipse(this.position.x,this.position.y,1);
}
//パーティクルが端に到達した時の処理
checkEdges(){
if(this.position.x>p.width){
this.position.x=0;
}
if(this.position.x < 0){
this.position.x=p.width;
}
if(this.position.y>p.height){
this.position.y=0;
}
if(this.position.y < 0){
this.position.y=p.height;
}
}
}
};
new p5(sketch2, "container2");
galleryページへ戻る